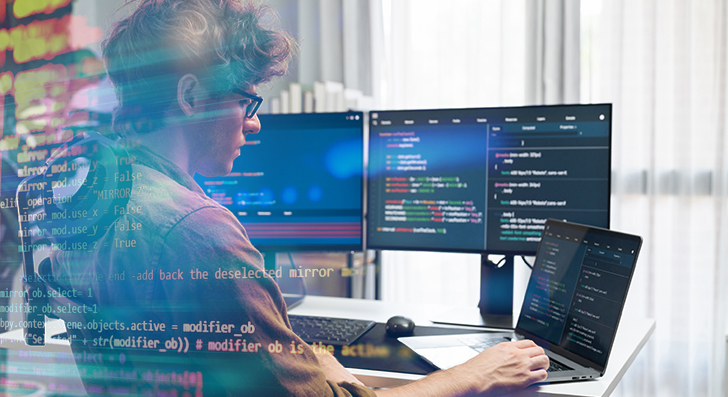
Scalability usually means your application can handle advancement—far more consumers, more details, and a lot more site visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and practical tutorial that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be element within your prepare from the start. Several purposes fall short every time they expand speedy for the reason that the original style and design can’t deal with the additional load. As a developer, you must think early about how your process will behave under pressure.
Get started by creating your architecture being flexible. Keep away from monolithic codebases where by every thing is tightly linked. In its place, use modular design and style or microservices. These styles break your app into scaled-down, unbiased components. Every single module or company can scale on its own without having impacting the whole program.
Also, contemplate your databases from working day 1. Will it need to have to manage one million users or simply 100? Pick the ideal kind—relational or NoSQL—depending on how your knowledge will improve. Approach for sharding, indexing, and backups early, even if you don’t require them however.
A different vital point is to avoid hardcoding assumptions. Don’t create code that only functions below existing problems. Think of what would transpire if your person foundation doubled tomorrow. Would your application crash? Would the databases slow down?
Use style and design styles that guidance scaling, like concept queues or occasion-driven systems. These assist your app handle more requests without getting overloaded.
Whenever you Develop with scalability in mind, you're not just preparing for fulfillment—you might be lessening future headaches. A perfectly-prepared process is simpler to take care of, adapt, and increase. It’s superior to get ready early than to rebuild later.
Use the proper Databases
Picking out the proper database is usually a important A part of building scalable apps. Not all databases are created the identical, and utilizing the Erroneous one can slow you down or maybe lead to failures as your application grows.
Begin by understanding your facts. Could it be highly structured, like rows in a very table? If yes, a relational databases like PostgreSQL or MySQL is an efficient match. They're potent with associations, transactions, and regularity. Additionally they support scaling approaches like study replicas, indexing, and partitioning to take care of a lot more targeted visitors and facts.
Should your details is much more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling large volumes of unstructured or semi-structured knowledge and will scale horizontally much more quickly.
Also, think about your read through and write patterns. Will you be doing a great deal of reads with fewer writes? Use caching and browse replicas. Will you be handling a hefty publish load? Look into databases that will cope with high create throughput, as well as event-based mostly knowledge storage units like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You might not need Superior scaling characteristics now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to hurry up queries. Keep away from unwanted joins. Normalize or denormalize your details depending on your access designs. And constantly keep an eye on databases functionality while you increase.
Briefly, the appropriate databases is dependent upon your application’s construction, velocity desires, And just how you be expecting it to improve. Acquire time to choose properly—it’ll conserve plenty of difficulty later.
Improve Code and Queries
Quick code is key to scalability. As your application grows, just about every modest delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop effective logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away everything needless. Don’t choose the most advanced Resolution if an easy 1 works. Maintain your functions shorter, centered, and easy to check. Use profiling resources to find bottlenecks—destinations in which your code takes too very long to run or takes advantage of excessive memory.
Next, check out your database queries. These generally slow points down greater than the code alone. Ensure Every single question only asks for the information you truly want. Stay clear of Pick *, which fetches click here all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, In particular across huge tables.
For those who discover the exact same data currently being asked for again and again, use caching. Retailer the effects temporarily making use of tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Make sure to exam with large datasets. Code and queries that function fantastic with one hundred data could possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Keep the code limited, your queries lean, and use caching when needed. These steps assist your application remain smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more website traffic. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid keep your app speedy, secure, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server undertaking each of the function, the load balancer routes customers to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to setup.
Caching is about storing data quickly so it may be reused quickly. When people request the same information and facts once again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly accessibility.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t alter generally. And usually ensure that your cache is updated when knowledge does improve.
In a nutshell, load balancing and caching are uncomplicated but potent instruments. Together, they help your application tackle much more end users, continue to be rapidly, and Get better from issues. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need applications that let your app expand simply. That’s where by cloud platforms and containers come in. They give you overall flexibility, cut down set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to purchase hardware or guess potential capability. When targeted traffic boosts, you may add more resources with just a few clicks or immediately utilizing auto-scaling. When visitors drops, you'll be able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You can focus on setting up your application in place of running infrastructure.
Containers are A further critical Device. A container deals your app and all the things it really should operate—code, libraries, options—into 1 device. This causes it to be straightforward to move your application amongst environments, out of your laptop into the cloud, with no surprises. Docker is the preferred Device for this.
When your application employs numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it instantly.
Containers also make it straightforward to independent aspects of your app into services. You may update or scale sections independently, which can be perfect for functionality and trustworthiness.
In a nutshell, utilizing cloud and container instruments indicates you could scale quickly, deploy conveniently, and Recuperate immediately when difficulties materialize. If you'd like your application to develop devoid of limits, start off making use of these instruments early. They save time, lessen hazard, and enable you to continue to be focused on creating, not repairing.
Monitor Every little thing
When you don’t monitor your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your application is carrying out, location problems early, and make far better selections as your application grows. It’s a key A part of constructing scalable devices.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just monitor your servers—monitor your app way too. Control how much time it will require for buyers to load internet pages, how frequently faults materialize, and where by they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a support goes down, you ought to get notified promptly. This can help you correct concerns quickly, frequently prior to users even see.
Checking is additionally helpful whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again before it results in true injury.
As your application grows, website traffic and info boost. With out checking, you’ll skip indications of difficulties until finally it’s much too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big providers. Even tiny applications require a robust foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you can Create applications that develop efficiently without breaking under pressure. Start out little, Consider significant, and Develop clever.